C#生成并发送手机验证码
目前用手机号+验证码的登录方式日渐广泛,那么C#如何实现发送手机验证码呢?
首先肯定要接入第三方的短信平台,因为这种发短信的服务并不是我们个人或者普通企业能接触到的,今天案例中所用到的短信平台是容联云通讯,其实在实际开发过程中我们自己写的代码是比较少的,一般服务商都会集成好接口,然后我们直接调用就可以了。
首先要下载容联云的C#功能集成类:点击下载(评论留邮箱)
然后创建一个短信验证码发送的实现类PhoneMessage.cs,这个类和上面那个功能集成类要在同一命名空间下。
传入参数有三个:手机号码(多个用,隔开)、短信模板ID(在容联云后台获取)、内容(要求为string数组)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace VehicleInterface.Web
{
public class PhoneMessage
{
/// <summary>
/// API(短信接收号码,短信模版ID,内容数据)
/// </summary>
/// <param name="phoneNumber">短信接收号码</param>
/// <param name="templateId">短信模版ID</param>
/// <param name="context">内容数据</param>
public void GoMessage(string phoneNumber, string templateId, string[] context)
{
string ret = null;
CCPRestSDK api = new CCPRestSDK();
bool isInit = api.init("app.cloopen.com", "8883");//(短信验证码接口地址,端口)
api.setAccount("自己申请","自己申请");//(主账号,主账号令牌)
api.setAppId("自己申请");//应用ID
try
{
if (isInit)
{
Dictionary<string, object> retData = api.SendTemplateSMS(phoneNumber, templateId, context);//(短信接收号码,短信模版ID,内容数据)
ret = getDictionaryData(retData);
}
else
{
ret = "初始化失败";
}
}
catch (Exception exc)
{
ret = exc.Message;
}
}
private string getDictionaryData(Dictionary<string, object> data)
{
string ret = null;
foreach (KeyValuePair<string, object> item in data)
{
if (item.Value != null && item.Value.GetType() == typeof(Dictionary<string, object>))
{
ret += item.Key.ToString() + "={";
ret += getDictionaryData((Dictionary<string, object>)item.Value);
ret += "};";
}
else
{
ret += item.Key.ToString() + "=" + (item.Value == null ? "null" : item.Value.ToString()) + ";";
}
}
return ret;
}
}
}
以上搞完之后我们就可以实现验证码的发送了。
PhoneMessage phoneMessage = new PhoneMessage();
string phone=“17700000000”;
Random rNum = new Random();
int num1 = rNum.Next(0, 9);
int num2 = rNum.Next(0, 9);
int num3 = rNum.Next(0, 9);
int num4 = rNum.Next(0, 9);
string[] numberCode = new string[1] { num1.ToString() + num2.ToString() + num3.ToString() + num4.ToString() };
phoneMessage.GoMessage(pNumber, "模板ID", numberCode);
多个手机号的话就用,隔开,17700000000,17700000001,........
日常附个图 。案例结束。继续码代码去咯。。
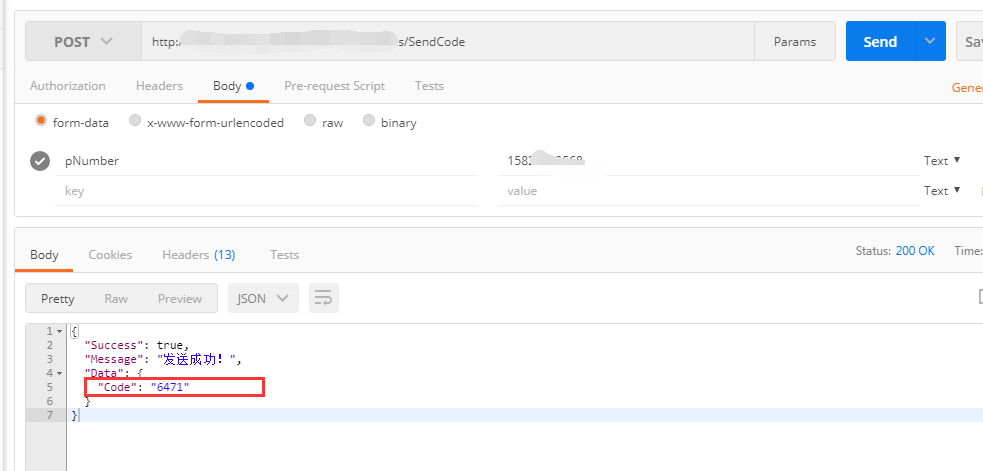
版权声明:
作者:兴兴
文章:C#生成并发送手机验证码
链接:https://www.networkcabin.com/original/1177
文章版权归本站所有,未经授权请勿转载。
作者:兴兴
文章:C#生成并发送手机验证码
链接:https://www.networkcabin.com/original/1177
文章版权归本站所有,未经授权请勿转载。
THE END